Stream a Recording
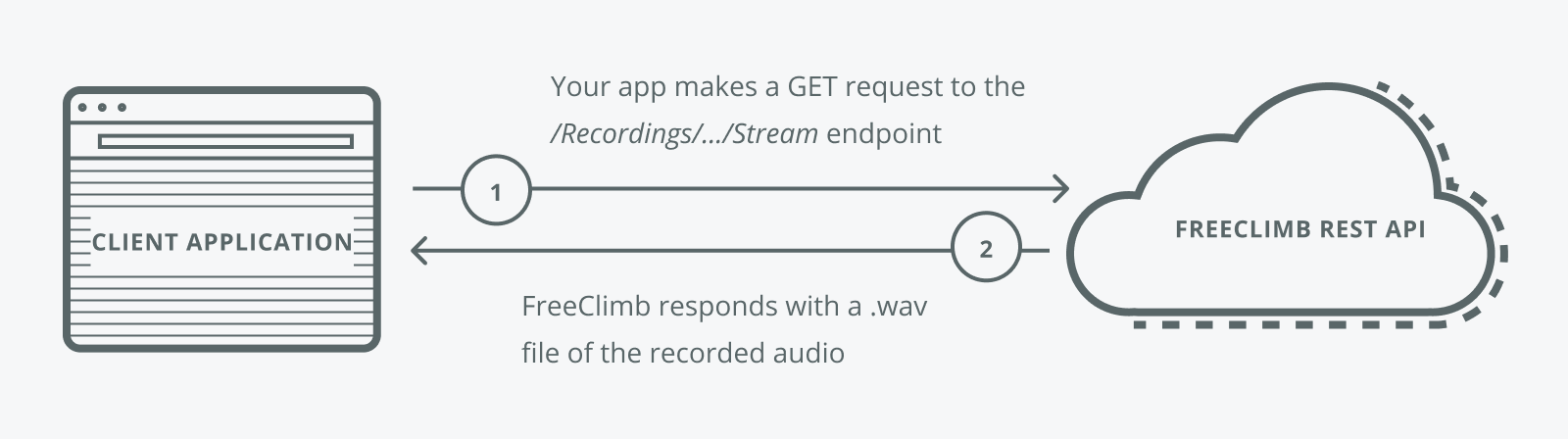
You're ready for this how-to guide if you've got the following:
A FreeClimb account
A registered application
A configured FreeClimb Number
Your tools and language installed
Node.js
Using the FreeClimb SDK an asynchronous request to stream a given recording can be initiated (recordingId
not provided for this example). Successful invocation will return a Node readable stream which may be read or redirected as needed.
Create your package.json file and save in the root directory of your project:
{
"name": "node-stream-a-recording-how-to-guide",
"version": "1.0.0",
"license": "MIT",
"dependencies": {
"@freeclimb/sdk": "^3.8.0",
"dotenv": "^8.1.0"
}
}
Install the package by running the following in the command line/terminal:
yarn install
Example code:
require('dotenv').config()
const freeclimbSDK = require('@freeclimb/sdk')
const accountId = process.env.ACCOUNT_ID
const apiKey = process.env.API_KEY
const configuration = freeclimbSDK.createConfiguration({ accountId, apiKey })
const freeclimb = new freeclimbSDK.DefaultApi(configuration)
const recordingId = 'YOUR_RECORDING_ID'
freeclimb.streamARecordingFile(recordingId)
.then(({ data }) => {
console.log(`Received ${data.length} bytes of data.`)
}).catch(err => {
console.log(err)
})
Java
To initiate any interaction with FreeClimb a FreeClimbClient
object must be created. Using the RecordingsRequester
created upon successful creation of the FreeClimbClient
a synchronous request to stream a given recording can be initiated. Successful invocation will return a KnownSizeInputStream
which may be read or redirected as needed. The KnownSizeInputStream
is a special variant on the standard Java InputStream
that exists in the FreeClimb SDK where the size of the underlying data is known.
Create your build.gradle file and save it to the root directory in your project:
/*
* This file was generated by the Gradle 'init' task.
*
* This is a general purpose Gradle build.
* Learn how to create Gradle builds at https://guides.gradle.org/creating-new-gradle-builds
*/
buildscript {
repositories {
mavenCentral()
maven { url 'https://jitpack.io' }
}
}
apply plugin: 'java'
apply plugin: 'eclipse'
apply plugin: 'idea'
repositories {
mavenCentral()
maven { url 'https://jitpack.io' }
}
sourceCompatibility = 1.8
targetCompatibility = 1.8
dependencies {
testCompile "junit:junit"
compile 'com.github.FreeClimbAPI:FreeClimb-Java-SDK:3.0.0'
}
jar {
manifest {
attributes 'Main-Class': 'stream_a_recording.StreamRecording'
}
}
sourceSets {
main {
java {
srcDirs = ['src'] // changed line
}
}
}
Build the file by running the following in your terminal/command line:
gradle build
Example code:
import com.vailsys.freeclimb.api.FreeClimbClient;
import com.vailsys.freeclimb.KnownSizeInputStream;
import com.vailsys.freeclimb.api.FreeClimbException;
FreeClimbClient client;
KnownSizeInputStream stream;
try {
// Create FreeClimbClient object
// accountId & apiKey can be found under API credentials on the FreeClimb Dashboard
client = new FreeClimbClient(accountId, apiKey);
/*
* Make the request for the recording. Receiving an InputStream in
* return which can be used to stream the recording.
*/
stream = client.recordings.stream(recordingId);
}
catch(FreeClimbException pe) {
System.out.println(pe.getMessage());
}
C#
To initiate any interaction with FreeClimb a FreeClimbClient
object must be created. Using the RecordingsRequester
created upon successful creation of the FreeClimbClient
a synchronous request to stream a given recording can be initiated. Successful invocation will return an IO stream which may be read or redirected as needed. In the sample the result is streamed via a FileStreamResult
action.
Imports used:
using com.freeclimb.api;
Example code:
try {
// Create FreeClimbClient object
FreeClimbClient client = new FreeClimbClient(FreeClimbAccountId, FreeClimbApiKey);
// Invoke get method with AudioReturn format of Stream to obtain IO stream of audio
Stream stream = client.getRecordingsRequester.stream(RecordingId);
}
catch (FreeClimbException ex) {
// Exception throw upon failure
}
Updated 19 days ago